Component Options
The component options can be initializated by sending this as a object to the class creator or by data-*
. To see how to use the data-*
, see the section Setting options from data-* in Getting Started.
The component set in the creator, has support for the below options:
- width
- height
- sliderClass
- sliderContent
- onResize (callback)
- onSliderMove (callback)
Component size
The component size can be set by thewidth
and height
property in options. The width
and height
properties accept number
, percent
or the aspect ratio for the height
. Some examples of use this properties is below:
Fixed Width and Height
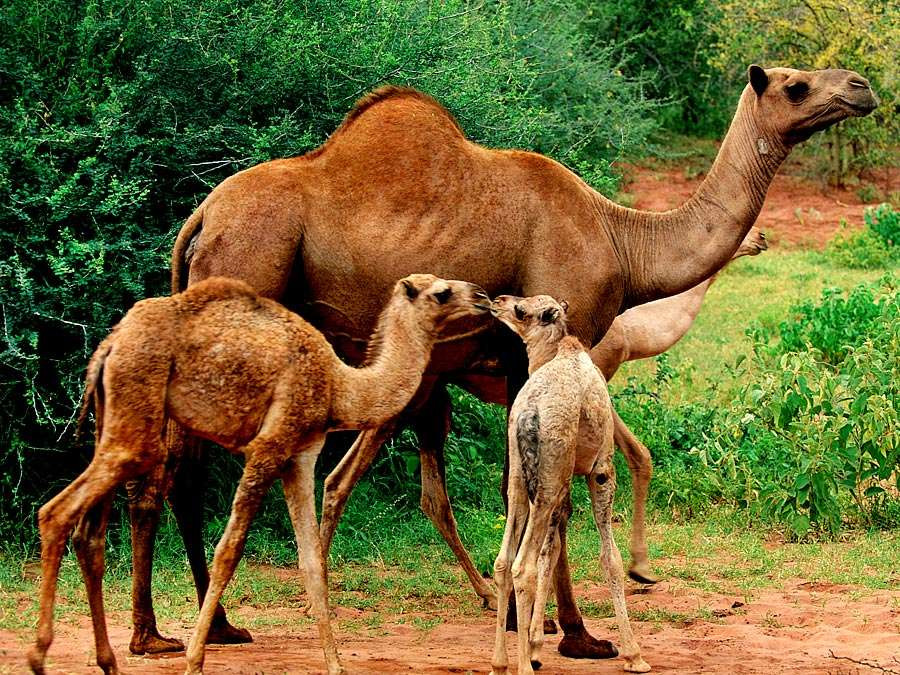
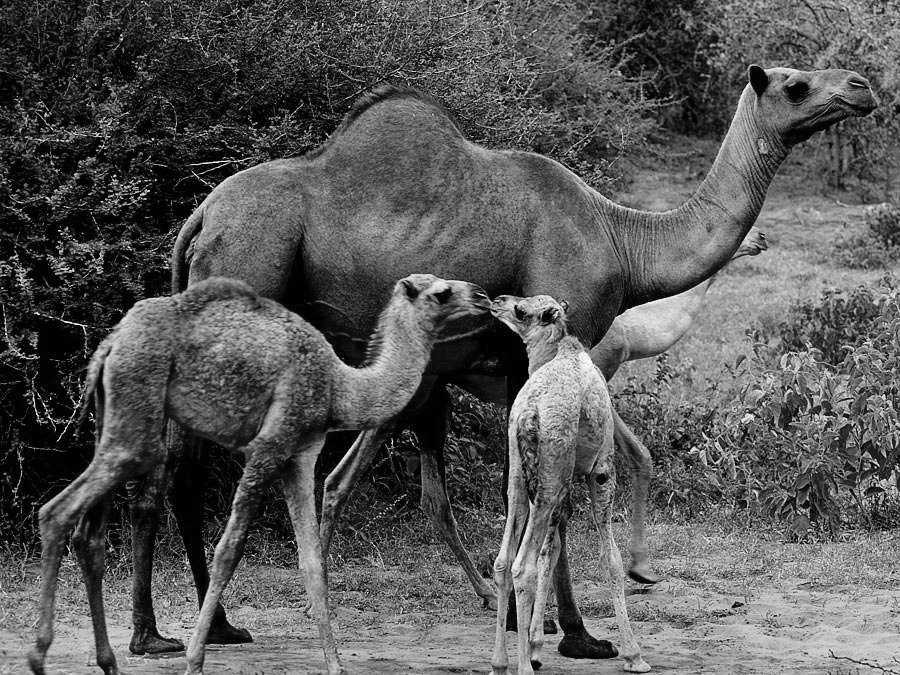
<div class="image-compare" data-width="300" data-height="300">
<img src="./images/comparer-1.jpg">
<img src="./images/comparer-2.jpg">
</div>
Or (in constructor)...
var el = document.getElementById('my-image-compare');
var comp = new ImageCompare(el, {
width: 300,
height: 300
});
Fixed Width and Proportional Height
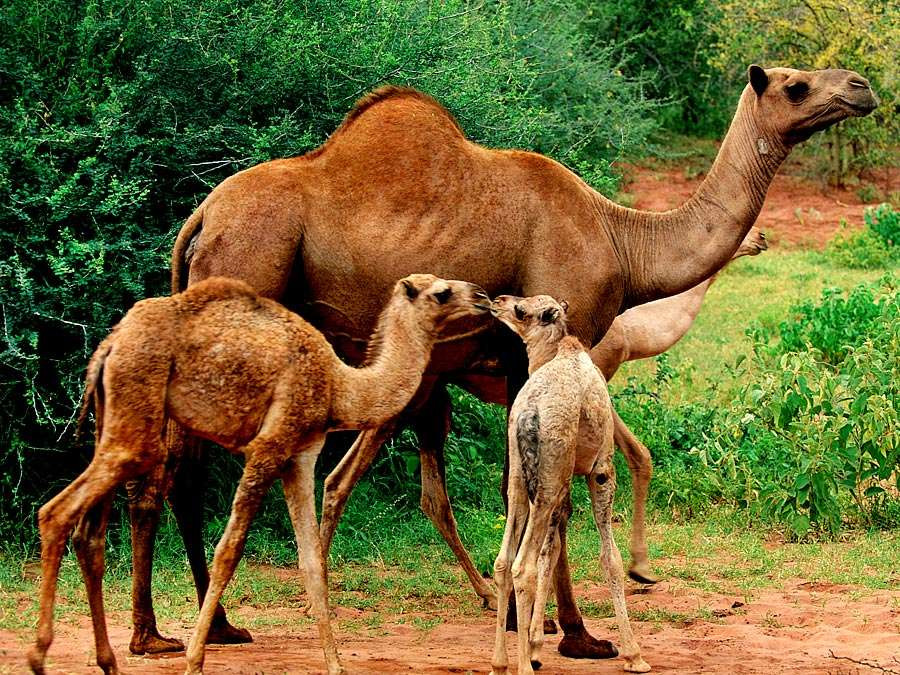
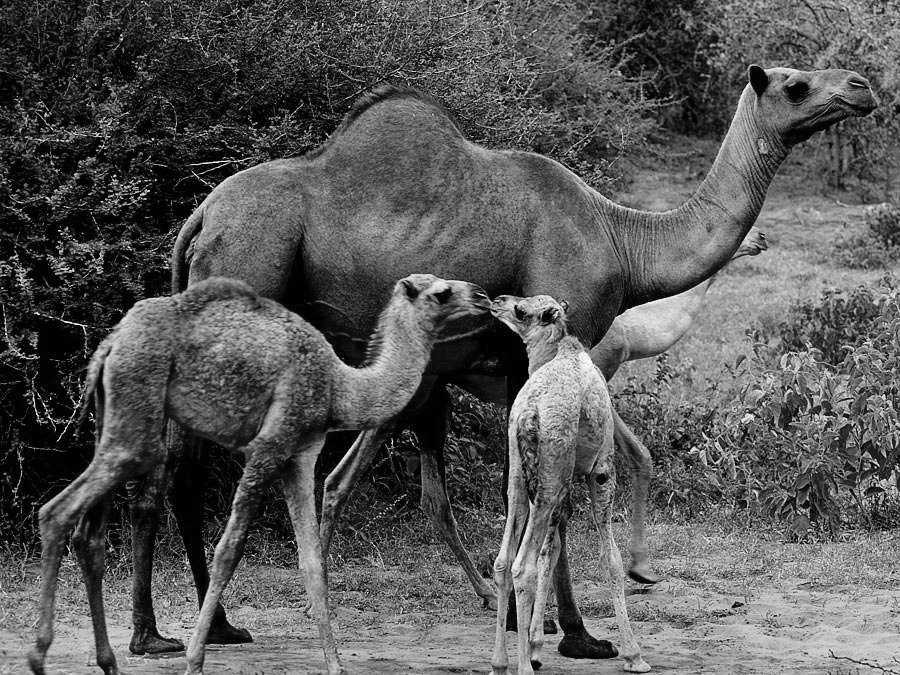
<div class="image-compare" data-width="300" data-height="auto">
<img src="./images/comparer-1.jpg">
<img src="./images/comparer-2.jpg">
</div>
Or (in constructor)...
var el = document.getElementById('my-image-compare');
var comp = new ImageCompare(el, {
width: 300,
height: 'auto'
});
Fixed Width and Proportional Height
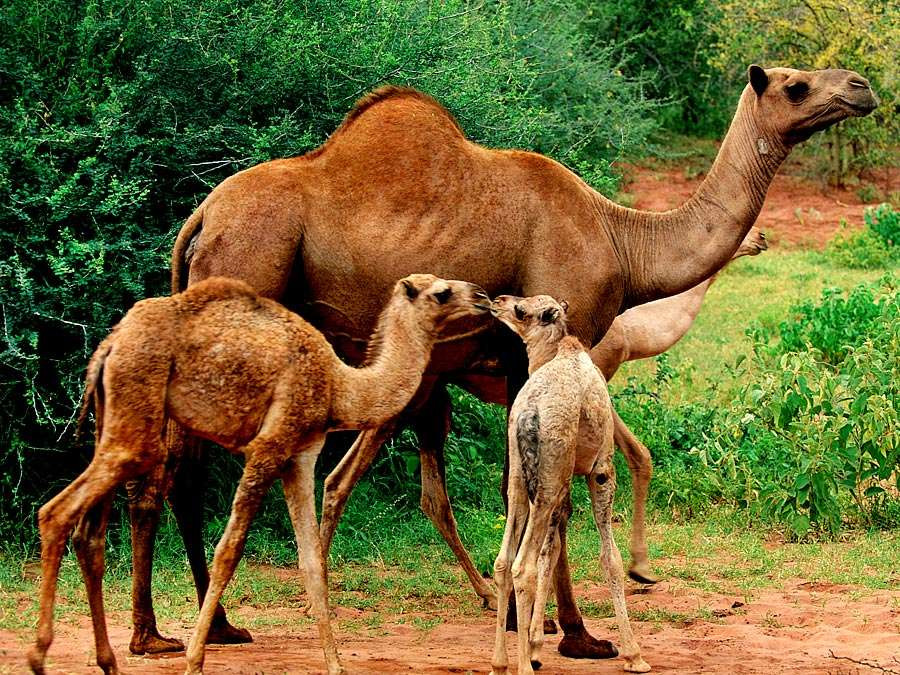
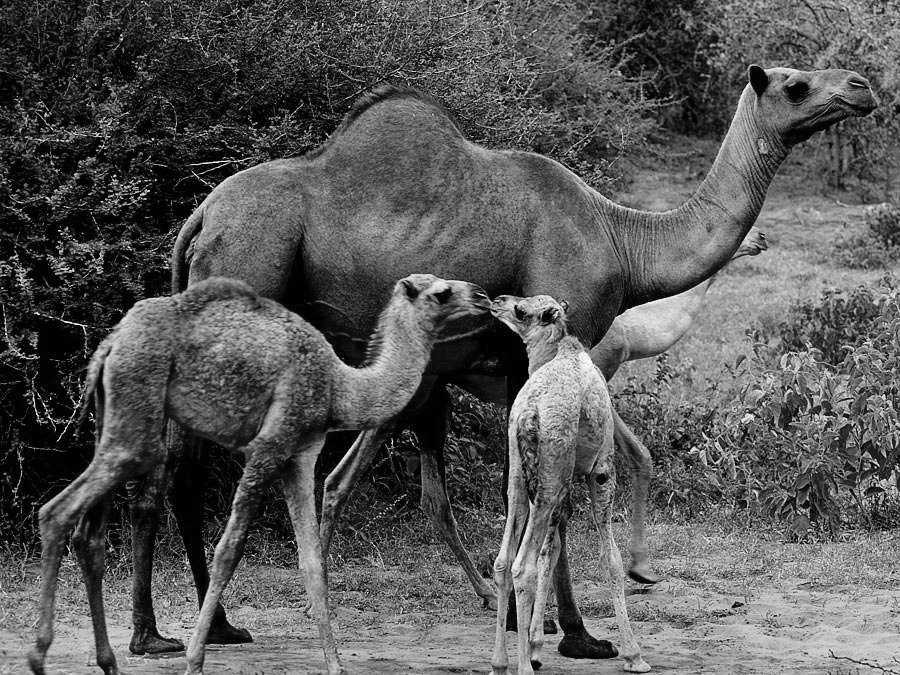
<div class="image-compare" data-width="300" data-height="4/3">
<img src="./images/comparer-1.jpg">
<img src="./images/comparer-2.jpg">
</div>
Or (in constructor)...
var el = document.getElementById('my-image-compare');
var comp = new ImageCompare(el, {
width: 300,
height: '4/3'
});
Note: for the proportional height, the accepted values by the component is auto
, 4/3
, 21/9
and 16/9
.
Relative Width and Proportional Height
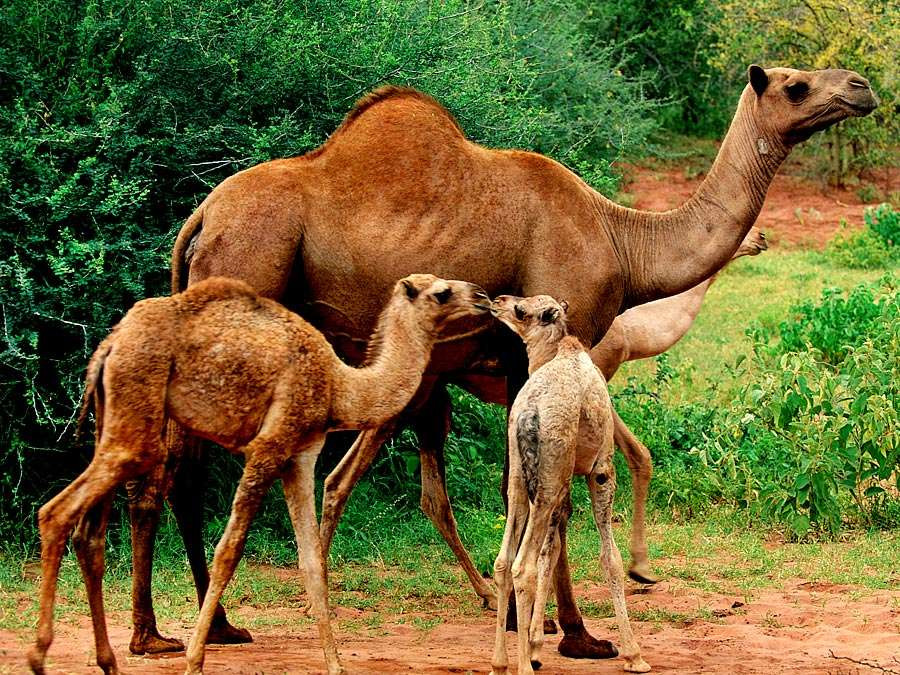
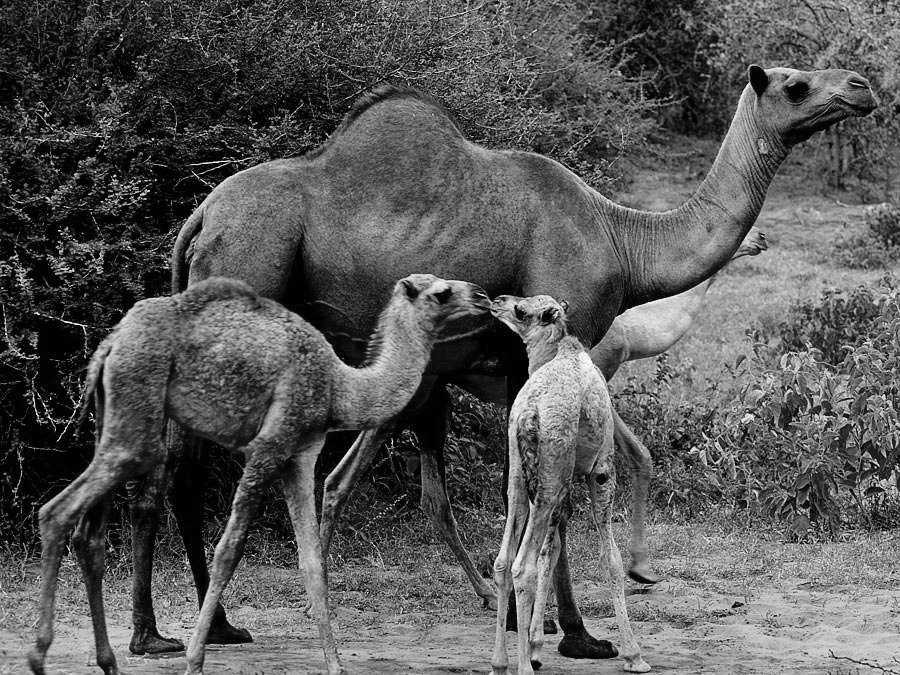
<div class="image-compare" data-width="70%" data-height="4/3">
<img src="./images/comparer-1.jpg">
<img src="./images/comparer-2.jpg">
</div>
Or (in constructor)...
var el = document.getElementById('my-image-compare');
var comp = new ImageCompare(el, {
width: '70%',
height: '4/3'
});
sliderClass
The sliderClass
property in options is used to set classes to the slider in the image comparer. This property accepts string
or array
, if this is a string
, this is added to classList
(note: if this is an string
, then you can set various classes by separating each by a whitespace, like "blue my-slider"
) property of the slider, but if is a array
, each element in the array
is added to classList
of the slider. You can use this to set the color or format, like a circle, in the slider, see some examples:
Materialize Red Circle
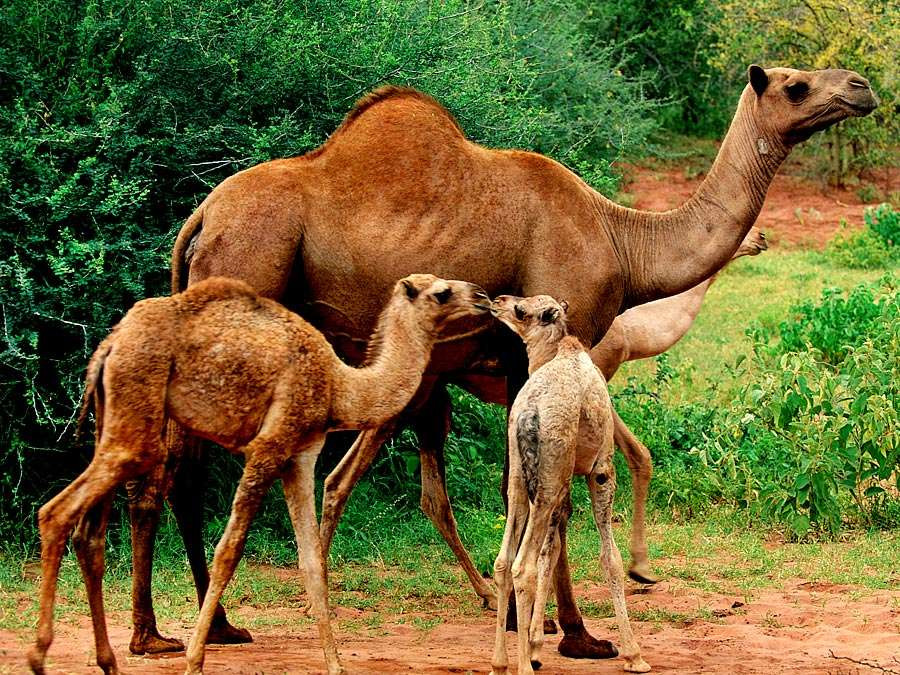
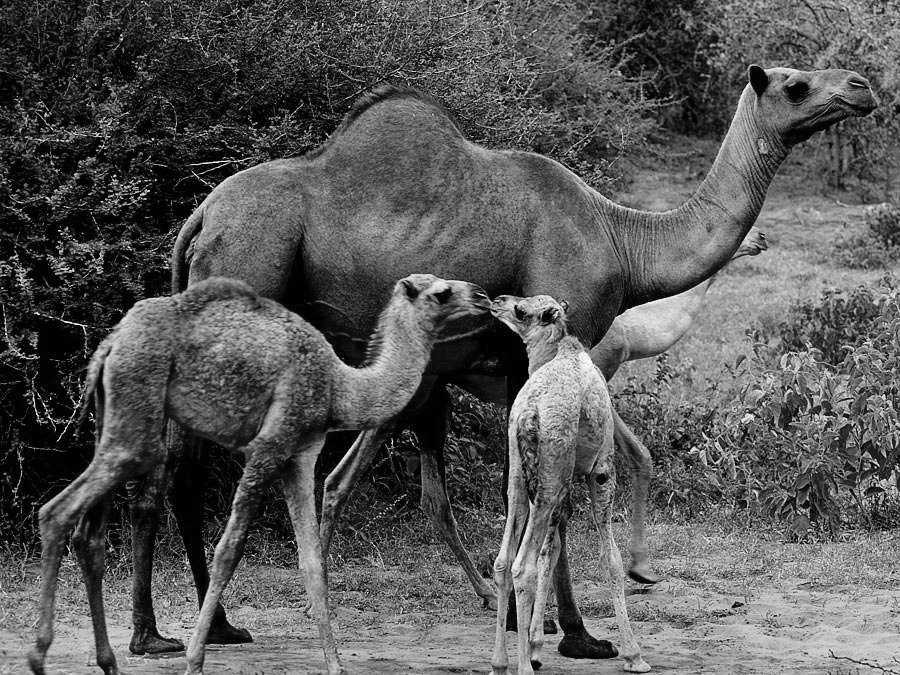
<div class="image-compare" data-slider-class="circle red">
<img src="./images/comparer-1.jpg">
<img src="./images/comparer-2.jpg">
</div>
Or (in constructor)...
var el = document.getElementById('image-compare-option-red-circle');
var comp = new ImageCompare(el, {
sliderClass: ['red', 'circle']
});
Or (in constructor)...
var el = document.getElementById('image-compare-option-red-circle');
var comp = new ImageCompare(el, {
sliderClass: 'red circle'
});
sliderContent
The sliderContent
property in options is used to set the content of the slider, for example if you need to add an icon in the slider. See an example:
Material-Icon
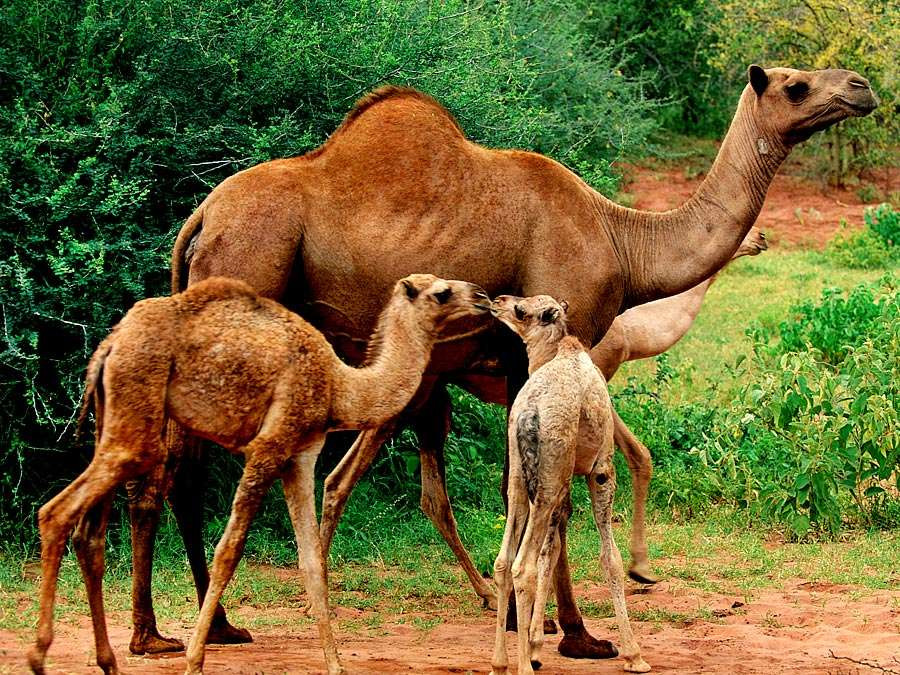
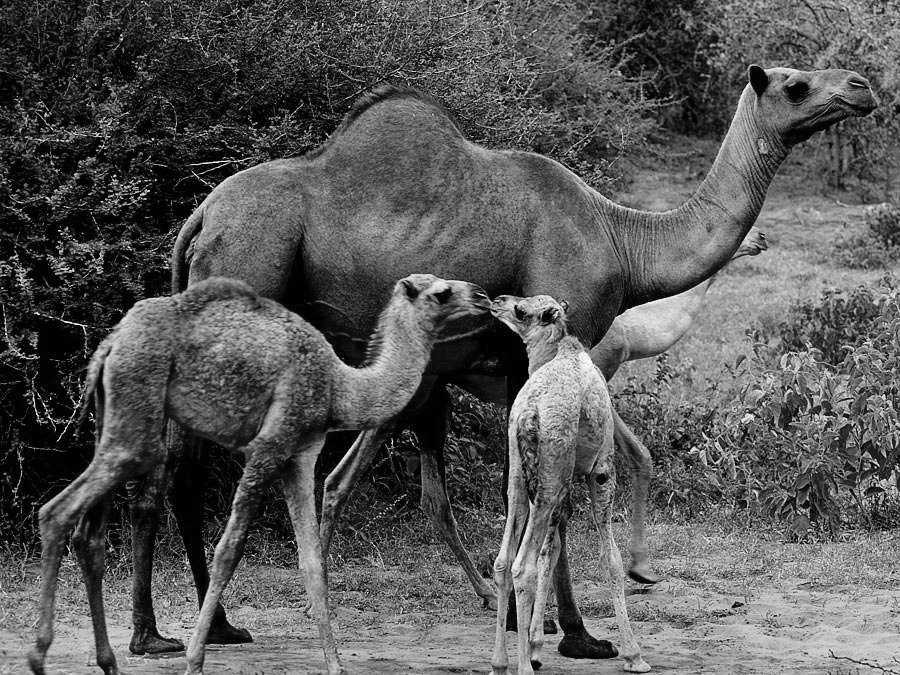
<div class="image-compare"
data-width="70%"
data-slider-class="red circle icon-to-center noselect"
data-slider-content="<i class='material-icons'>keyboard_tab</i>"
>
<img src="./images/comparer-1.jpg">
<img src="./images/comparer-2.jpg">
</div>
Or (in constructor)...
var el = document.getElementById('image-compare-slider-content');
var comp = new ImageCompare(el, {
width: '70%',
sliderClass: ['red', 'circle', 'icon-to-center', 'noselect'],
sliderContent: '<i class="material-icons">keyboard_tab</i>',
});
And the css of used classes:
.icon-to-center {
display: flex;
align-items: center;
justify-content: center;
}
.noselect {
-webkit-touch-callout: none; /* iOS Safari */
-webkit-user-select: none; /* Safari */
-khtml-user-select: none; /* Konqueror HTML */
-moz-user-select: none; /* Old versions of Firefox */
-ms-user-select: none; /* Internet Explorer/Edge */
user-select: none; /* Non-prefixed version, currently
supported by Chrome, Opera and Firefox */
}
onResize
The onResize
callback has the arguments (width, height, element)
.
var el = document.querySelector('#my-image-compare');
var comp = new ImageCompare(el, {
width: '70%',
onResize: function(w, h, element) {
console.log("resized");
}
});
onSliderMove
TheonSliderMove
callback has the arguments (x, left_slider_pos, slider_element)
. This callback is executed when the slider is moved.
var el = document.querySelector('#my-image-compare');
var comp = new ImageCompare(el, {
width: '100%',
onSliderMove: function(x, pos, slider) {
console.log("moved");
}
});